
Here I am with my first experiment on this blog.
I was trying to get a videowall effect by displaying many times the same video tiled on X rows and columns.
The bad one…
The logic says to create N*N Video Class instances and position them dinamically with a double for loop on rows and columns.
Alternatively we can speed up things by using the FLVPlayback component so we don’t need to manage connections and NetStream objects.
As I expected that couldn’t be that simple. Just with 10*10 (100 for non-math guys ) wall the frame rate is fast dropping and audio and video desynch issues appear. Another point is that you have to manually mute all FLVPlayback/Video instances except one for having one single audio playback.
Here is the “bad” code:
/*
SUMMARY:
This example will try to create a video wall instancing many times the FLVPlayback component with the same video source.
Results are very poor performances and audio sync issues.
*/
import fl.video.FLVPlayback;
/* set the number of horizontal and vertical tiles
a matrix of tiles*tiles instances will be created
try with very low numbers to avoid crashes.
A value of 10 will produce (10*10=) 100 instances of the FLVPlayback on the stage
*/
var tiles:uint=4;
// get stage size for tile size calculating
var sw:Number=stage.stageWidth;
var sh:Number=stage.stageHeight;
// calculate the size of a single tile
var vw:Number=sw/tiles;
var vh:Number=sh/tiles;
// perform a double cicle on rows and columns
for (var i:uint=0; i
If you use FLVPlayback as I do in the above code just remember to drop the component in the library before compiling the movie or flash will not be able to attach it dinamically.
And here is the (almost) working example.
As you can see videos are not perfectly synchronized and just raise a little the tiles number to make everything laggy.
...and the good one
Ok, now the better idea in another picture and few words:
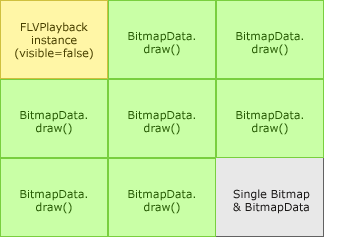
- Add a single FLVPlayback instance
- create e bitmapdata and a bitmap to display it
- add a frame based event to apply the draw() method of the bitmapdata to the video instance N*N times at everyframe with a translation matrix to set the position of every tile.
- add the bitmap to the stage and hide the FLVPlayback instance.
- Enjoy
I really couldn't believe how the new AS3 bitmap engine can perform well once again. I have added a numeric stepper to let you dinamically change the tiles number and test it yourself. I set the limit to 50*50 (2500!) tiles and on my old Athon XP 3200 it just shows a little of audio stuttering when reaching that limit.
Here is the well working example,
and here comes the code:
/*
SUMMARY:
This example will use a single bitmap and the bitmapdata draw() method to render everyframe the video content as texture
*/
import fl.video.FLVPlayback;
import fl.video.VideoScaleMode;
import flash.display.Bitmap;
import flash.display.BitmapData;
import flash.geom.Matrix;
import fl.controls.NumericStepper;
// get stage size for tile size calculating
var sw:Number=stage.stageWidth;
var sh:Number=stage.stageHeight;
var tiles:uint;
var vw:Number;
var vh:Number;
// create a single instance of the FLVPlayback component
var video:FLVPlayback=new FLVPlayback();
video.source="onepiece.flv";
video.scaleMode=VideoScaleMode.EXACT_FIT;
video.seek(7);
// add to stage the newly created FLVPlayback instance
addChild(video);
video.visible=false;
// create the destination bitmap/bitmapdata
var bdata:BitmapData=new BitmapData(sw, sh, false, 0xeeeeee);
var bitmap:Bitmap=new Bitmap(bdata);
addChild(bitmap);
// perform a double cicle on rows and columns
function setTiles():void {
// get the tiles number from the NumericStepper value
tiles=stepper.value;
// calculate the size of a single tile
vw=sw/tiles;
vh=sh/tiles;
video.setSize(vw, vh);
}
addEventListener(Event.ENTER_FRAME, onframe);
function onframe(event:Event):void {
for (var i:uint=0; i
Hope you liked my first actionscript post.
Edit: I didn't forget the beginBitmapFill method, but the project started a little different (not all the tiles was identical) and so I left the manual loop. In a few times I'll post the beginBitmapFill version for performance comparison.
